Here is a small example how to create a textbox validation mechanism. In this example we will create a textbox and a button to validate the value of textbox, and we will write some javascript for validation. The textbox and Validate button should look like following:
Our aim is:
if some one click on validate button without typing anything in textbox it should display a message as following.
And finally when someone type a pure number then it should allow the number and display following.
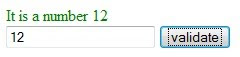
So here is the complete code of javascript and html.
Create a file indx.html and type following code in it.
<html>
<head>
<script type="text/javascript">
var str ='0123456789';
function myfunction()
{
var textBox = document.getElementById("numtxt");
var msg = document.getElementById('msg');
if(textBox.value.length==0)
{
msg.style.color = 'red';
msg.innerHTML = 'Please enter something in textbox';
return;
}
for(var i=0; i<textBox.value.length; i++)
{
if(str.indexOf(textBox.value.charAt(i))==-1)
{
msg.style.color = 'red';
msg.innerHTML = 'This is not a number';
return;
}
}
msg.style.color = 'green';
msg.innerHTML = 'It is a number '+textBox.value;
}
</script>
</head>
<body>
<form>
<div id="msg" bgcolor='red'>Msg Will Display Here</div>
<input type="text" id="numtxt" name="salary" />
<input type="button" value="validate" onclick="myfunction()"/>
</form>
</body>
</html>
Now open index.html in a browser and see magic.
No comments:
Post a Comment